A Brief overview of Arrays and Linked Lists
Arrays and Linked Lists can be methods to store data on the computer arrays are like a row of boxes that are numbered. They help you organize things and each box is assigned its own number. They are useful for finding items by their numbers.
They have an exact size, meaning that you determine the size of boxes before you begin linked lists are like chains that hold a particular item and are aware of which box it is.
They’re ideal for removing or adding items easily, but finding things may take longer since they start from the beginning and then follow the chain. In simplest terms, they’re like an ordered row of boxes that makes it easy to locate things as linked lists function like an array of boxes, where the process of finding something can take a bit more time.
What is Arrays?
Arrays allow you to keep a lot of things together, for example, the numbered boxes. Each box is a container for things (like words or numbers) and comes with its own number. Imagine the rows of baskets each one labeled with a number between 0 2, etc.
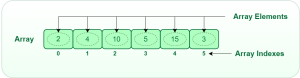
You can place different items into the baskets, and each is placed in a particular arrangement. This makes it simple to locate items quickly as you know the number of the basket.
Arrays are the same size as a basket, so you have to determine the number of baskets you’ll need in the beginning and can’t modify the size in the future. Arrays can be useful in organizing your things and finding things quickly by utilizing their number of places.
What is Linked Lists?
Linking lists are a method to organize items, similar to the chain of connected boxes. Each box contains the item it is attached to and points to the next one that is in the same chain. Imagine a chain of treasure chests with each one having something inside as well as a clue to where to find the next.

Like the arrays of linked lists, they do not require an exact order. Moving chests around is simple because each chest is aware of which one is next. But, finding the right chest could take longer as you might have to start at the beginning and then look for clues.
Linked lists offer the flexibility of adding or removing things in a hurry, however, the search for a specific item may require a bit longer when compared to arrays because of the search in a sequential manner.
Key Difference Between Arrays and Linked Lists
Here’s a concise comparison chart between arrays and linked lists:
Aspect | Arrays | Linked Lists |
---|---|---|
Memory Allocation | Contiguous block in memory | Non-contiguous, linked by pointers |
Size | Fixed size | Dynamic size, easily resizable |
Order | Sequential order | No inherent order, elements linked |
Access Time | Quick access via index | Slower access, requires traversal from the beginning |
Insertion | Costly for inserting elements | Efficient insertion anywhere in the list |
Deletion | Costly due to shifting elements | Efficient deletion, no shifting needed |
Flexibility | Limited flexibility | High flexibility in adding/removing elements |
Implementation | Easier implementation | More complex due to pointers |
Use Case | Fixed-size data, fast access needs | Dynamic data, frequent insertions/deletions |
Advantages and Limitations
Arrays:
Advantages:
- Quick Access: The elements of arrays are accessible quickly by their index, allowing for the ability to access them at any time (O(1)).
- Sequential Access: arrays work great to store and retrieve sequenced data, such as grids or lists.
- Simple Implementation: Arrays are simple to use and implement for programming in languages.
Limitations:
- Fixed Size: The size of arrays is determined at the beginning of their life and cannot be dynamically changed in size without creating an array from scratch.
- Inefficient Insertion: Inserting or removing elements within arrays may be unfast and slow, specifically when large arrays are involved since it might require shifting elements.
Linked Lists:
Advantages:
- Dynamic Size: Lists linked can expand or shrink in a matter of minutes to allow the dynamic allocation of memory.
- Efficient Insertion and Removal: Inserting or removing items from the linked list is effective since it doesn’t require shifting elements, it’s just about changing the pointers.
- Flexibility: Different types of linked lists (singly circular, doubly,) provide different benefits in various applications.
Limitations:
- Slow access: Accessing elements in linked lists is more difficult when compared to arrays as it requires traversing the list from the beginning.
- Additional Memory Cost: Lists that are linked use additional memory to store pointers, which increases memory overhead in comparison to arrays.
Summary
Arrays store data in a fixed-size, sequential manner, enabling quick access through indexes but with limitations on size flexibility and inefficient insertions/deletions. On the other hand, linked lists consist of nodes linked by pointers, allowing dynamic sizing and efficient element insertions or deletions.
Yet, linked lists have slow access times since they require navigating from the beginning. Arrays are suitable for fixed-size data and fast access requirements, whereas linked lists are more suited for changing data, putting the ease of deletion and insertion over the speed of access immediately.
The choice between them is based on the particular requirements of the system or program.